Declarively apply converter functions to class attributes.
Project description
Exert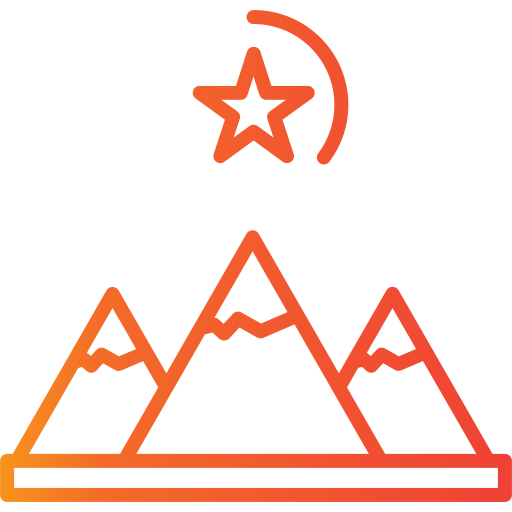
>> Declaratively apply converter functions to class attributes. <<
Installation
Install via pip:
pip install exert
Usage
Use this to declaratively apply arbitrary converter functions to the attributes of a class. For example:
from __future__ import annotations
from typing import Annotated
from exert import exert, Mark
@exert
class Foo:
a: Annotated[int, Mark(lambda x: x**2)]
b: Annotated[float, Mark(lambda x: x / 2)]
def __init__(self, a: int, b: float) -> None:
self.a = a
self.b = b
foo = Foo(2, 42.0)
print(foo.a) # prints 4
print(foo.b) # prints 21.0
Here, the lambda function tagged with Mark
is the converter.
Use with dataclasses
Dataclasses can also be used to avoid writing the initializer by hand. For example:
...
from dataclasses import dataclass
@exert
@datclasses
class Foo:
a: Annotated[int, Mark(lambda x: x**2)]
b: Annotated[float, Mark(lambda x: x / 2)]
foo = Foo(2, 42.0)
print(foo.a) # prints 4
print(foo.b) # prints 21.0
Apply multiple converters sequentially
Multiple converters are allowed. For example:
...
@exert
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x**2, lambda x: x**3)]
b: Annotated[float, Mark(lambda x: x / 2, lambda x: x / 3)]
foo = Foo(2, 42.0)
print(foo.a) # prints 64 [2**2=4, 4**3=64]
print(foo.b) # prints 7.0 [42.0/2=21.0, 21.0/3=7.0]
Here, the converters are applied sequentially. The result of the preceding converter is fed into the succeeding converter as input. You've to make sure that the number of the returned values of the preceding converter matches that of the succeeding converter.
Exclude annotated fields
If you don't wrap converters with Mark
, the corresponding field won't be transformed:
...
@exert
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x**2, lambda x: x**3)]
b: Annotated[float, lambda x: x / 2, lambda x: x / 3]
foo = Foo(2, 42.0)
print(foo.a) # prints 64 [2**2=4, 4**3=64]
print(foo.b) # prints 42.0 [This field was ignored]
Since the converters in field b
weren't tagged with Mark
, no conversion happened.
Apply common converters without repetition
Common converters can be applied to multiple fields without repetition:
...
@exert(converters=(lambda x: x**2,))
@dataclass
class Foo:
a: Annotated[int, None]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 4 [2**2=4]
print(foo.b) # prints 1764.0 [42.0**2=1764.0]
Apply common and marked converters together
You can apply a sequence of common converters and marked converters together. By default, the common converters are applied first and then the tagged converters are applied sequentially:
...
@exert(converters=(lambda x: x**2, lambda x: x**3))
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x / 100)]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 0.64 [2**2=4, 4**3=64, 64/100=0.64]
print(foo.b) # prints 5489031744.0 [42.0**2=1764, 1764**3=5489031744.0]
You can also, choose to apply the common converters after the tagged ones. For this,
you'll need to set the apply_last
parameter to True
:
...
@exert(
converters=(lambda x: x**2, lambda x: x**3),
apply_last=True,
)
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x / 100)]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 6.401e-11 [2/100=0.02, 0.02**2=0.004, 0.0004**3=6.401e-11]
print(foo.b) # prints 5489031744.0 [42.0**2=1764, 1764**3=5489031744.0]
Project details
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distributions
File details
Details for the file exert-0.3.1.tar.gz
.
File metadata
- Download URL: exert-0.3.1.tar.gz
- Upload date:
- Size: 4.9 kB
- Tags: Source
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0b9edef13d5a9921097d35939ad326c8804f7c19df0f7064ade425aaa0e4cb86 |
|
MD5 | a99b53c859883f759f6c4c0c23ae935d |
|
BLAKE2b-256 | 51cb213982b556718789c22f72f09a926bf7208109cd116c0480335c25e29c17 |
File details
Details for the file exert-0.3.1-cp310-cp310-musllinux_1_1_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp310-cp310-musllinux_1_1_x86_64.whl
- Upload date:
- Size: 87.2 kB
- Tags: CPython 3.10, musllinux: musl 1.1+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | e7a4a41e228afdf2354b3d64f33e22f9f616f70a6922f600bff25bef23383f3e |
|
MD5 | 4779665f3072f3420f63d54a404bf4f2 |
|
BLAKE2b-256 | 1785a38eb34f0a78a25025a03311bec0733f3ff9997c64da1cabb38a2862dbfe |
File details
Details for the file exert-0.3.1-cp310-cp310-musllinux_1_1_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp310-cp310-musllinux_1_1_i686.whl
- Upload date:
- Size: 87.0 kB
- Tags: CPython 3.10, musllinux: musl 1.1+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | b16f34054d27d7c6cc971b88cfcbb9d0e18ab0be1bc201104e14f5522c1dec05 |
|
MD5 | 91f7825adbecca2a1225f82fb9a122b6 |
|
BLAKE2b-256 | 2badf844d54280762ed44f97a03e156b823fe61e1198f8d997d3e84c81ab077f |
File details
Details for the file exert-0.3.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
- Upload date:
- Size: 91.3 kB
- Tags: CPython 3.10, manylinux: glibc 2.17+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | c3f26bc52faafa3a4d6935bc47461e6fa06012e00ac9e393e61d916be2e031c1 |
|
MD5 | abb570cd89e5ef50dc50c6a8d499f6e3 |
|
BLAKE2b-256 | 5a08679c12bb3def1afb0e925f2b69e04133458d9757837aebe84f9db5ebdef6 |
File details
Details for the file exert-0.3.1-cp310-cp310-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp310-cp310-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
- Upload date:
- Size: 90.2 kB
- Tags: CPython 3.10, manylinux: glibc 2.17+ i686, manylinux: glibc 2.5+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 33670b9bdcc3fae340ace858254d2518abf59d79714f2554c09426489f44b851 |
|
MD5 | cdbf40fc9286d197fcbfd093e3fdf6af |
|
BLAKE2b-256 | 8e38be57cd5439d95cfb1e84749b9881771618d6f05f59056a14619523192a52 |
File details
Details for the file exert-0.3.1-cp310-cp310-macosx_10_9_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp310-cp310-macosx_10_9_x86_64.whl
- Upload date:
- Size: 49.3 kB
- Tags: CPython 3.10, macOS 10.9+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 12d27b70f5a14a6987780a85cf4c270dbc087f11a05cda6eac4355a15f04d3d1 |
|
MD5 | 0c28f1603e9473c773c89c88234659d3 |
|
BLAKE2b-256 | 527b47527882d20973e3c844aff808bad6588edb2e6845bc3ad1eb41d4230050 |
File details
Details for the file exert-0.3.1-cp39-cp39-musllinux_1_1_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp39-cp39-musllinux_1_1_x86_64.whl
- Upload date:
- Size: 87.1 kB
- Tags: CPython 3.9, musllinux: musl 1.1+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 83520cd19a3c6944aafcf13372e9b0829acb997a13bbd398a73282327b217606 |
|
MD5 | fcb74bff78ad179af635a8fc6d6c9f55 |
|
BLAKE2b-256 | b2a79f64ca8f10453495558f8d331ae2665e123bc97ae98bb706528130be59d5 |
File details
Details for the file exert-0.3.1-cp39-cp39-musllinux_1_1_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp39-cp39-musllinux_1_1_i686.whl
- Upload date:
- Size: 86.8 kB
- Tags: CPython 3.9, musllinux: musl 1.1+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | f2ff2d54ba564ab27c4b61dd92af3b76f1d3c6e987b3b8791644118cd2cda979 |
|
MD5 | 5bb2ca50f9a63c8bf8dad78a89bb79bf |
|
BLAKE2b-256 | ac231f34e36058ac7ea6f2caf105f8dded1e5a3155b14b3e7f8fa926fe3a4055 |
File details
Details for the file exert-0.3.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
- Upload date:
- Size: 91.2 kB
- Tags: CPython 3.9, manylinux: glibc 2.17+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | a060bd6b32c3e4f4ca5c063ad9e2e5c5bbd40a20f4f52b8c5195d747ad1d9fcd |
|
MD5 | f73cc4aa3db058eee3f8061c34408443 |
|
BLAKE2b-256 | 92383ee50adcb5039a0d2d8e80b7ec8d12e412332b38c4f4ab9cf452817075c6 |
File details
Details for the file exert-0.3.1-cp39-cp39-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp39-cp39-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
- Upload date:
- Size: 90.1 kB
- Tags: CPython 3.9, manylinux: glibc 2.17+ i686, manylinux: glibc 2.5+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 700c49c466e36268df0ce210d95fa4a0fb31ec1762e9748135326a212f955a4c |
|
MD5 | a5761c6ef002e417a24e0966ef898699 |
|
BLAKE2b-256 | 0ab25112a122e6c59e3325db231a0c9ba93fb22385e70f0194da6721cc0b6ff7 |
File details
Details for the file exert-0.3.1-cp39-cp39-macosx_10_9_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp39-cp39-macosx_10_9_x86_64.whl
- Upload date:
- Size: 49.3 kB
- Tags: CPython 3.9, macOS 10.9+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 1fab360cd7f677a659640f1512fd21e0445d4e84dcf4f36d527a14fb479501fc |
|
MD5 | 75f9a985b8ca7a9aec5d25da81d02b89 |
|
BLAKE2b-256 | 12c050660a6e5fbc8ccebbdbf7b8881b90465611c11e90d054c57ef678fd7895 |
File details
Details for the file exert-0.3.1-cp38-cp38-musllinux_1_1_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp38-cp38-musllinux_1_1_x86_64.whl
- Upload date:
- Size: 86.5 kB
- Tags: CPython 3.8, musllinux: musl 1.1+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | e4212be1af9f02600b2a1b6932c539185e6eb35c2cc258f11d3152b59413155e |
|
MD5 | 7e5e86a8da36e0753894529f6b6a6fdf |
|
BLAKE2b-256 | b1867d4c7d8152b71ef0f4b11fb6571d456c80ef6246ef1e4c9cd308e53acc4d |
File details
Details for the file exert-0.3.1-cp38-cp38-musllinux_1_1_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp38-cp38-musllinux_1_1_i686.whl
- Upload date:
- Size: 86.2 kB
- Tags: CPython 3.8, musllinux: musl 1.1+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 8e58fd89d2b930d3b5822635ce01031e3f0293452d1f4f84416b4fdec08ec8d2 |
|
MD5 | 41fc38e663345c5738406c6beaabfb60 |
|
BLAKE2b-256 | 0f3afc2c16f20852ea88aed329f2643cd22e8641bdb85e168e583ca7ef580211 |
File details
Details for the file exert-0.3.1-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
- Upload date:
- Size: 90.1 kB
- Tags: CPython 3.8, manylinux: glibc 2.17+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | a23e3758024491ae039d2f438d39312852ba44e4ba3694b2391da0a99dfa3b2f |
|
MD5 | 281c4933b975bf09a215a25aad15b9f4 |
|
BLAKE2b-256 | cfa5d508bd2b8cada6f32d0f248caa13ed8c7d4277b95a04c0123f0e5f1872f7 |
File details
Details for the file exert-0.3.1-cp38-cp38-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp38-cp38-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
- Upload date:
- Size: 88.8 kB
- Tags: CPython 3.8, manylinux: glibc 2.17+ i686, manylinux: glibc 2.5+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 2f1bfb516dbbf7f9d0afe072c1ade5fcf565077860fff4fefc872db8a9f7efff |
|
MD5 | 5f1674036e765bc9be8ffcea869c8951 |
|
BLAKE2b-256 | 9614d04f7f34df9cf6936684f0553be748f74b0b9f2283d4152528e32c208046 |
File details
Details for the file exert-0.3.1-cp38-cp38-macosx_10_9_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp38-cp38-macosx_10_9_x86_64.whl
- Upload date:
- Size: 48.7 kB
- Tags: CPython 3.8, macOS 10.9+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0708db7b97ddcdbac068feefdc53d1bd4f7948a113d80ab835af2db10be6782e |
|
MD5 | 21af041b546dce22be91fcb2481dd120 |
|
BLAKE2b-256 | a505f8cad0ff95e75186c867fe785c8387f529f2f2811fee9f3ee44cbf699f75 |
File details
Details for the file exert-0.3.1-cp37-cp37m-musllinux_1_1_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp37-cp37m-musllinux_1_1_x86_64.whl
- Upload date:
- Size: 74.4 kB
- Tags: CPython 3.7m, musllinux: musl 1.1+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 92403ace54e78ca44b986d8ceb471c4bc46743d85658fce41795fda973681719 |
|
MD5 | 30bcb70998359a65bdc29de8fd9debe4 |
|
BLAKE2b-256 | cefd53ebad60df76db6fe429830f9248822c4e959a0bb3cb132ebb91e222c7ca |
File details
Details for the file exert-0.3.1-cp37-cp37m-musllinux_1_1_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp37-cp37m-musllinux_1_1_i686.whl
- Upload date:
- Size: 75.6 kB
- Tags: CPython 3.7m, musllinux: musl 1.1+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 18af65d14dbf6684e9e3b36caf08b55718e590a1a21c3be6ad40d4ab1d376abc |
|
MD5 | c6e5eaa2a8ded04df1a818f16bd791ce |
|
BLAKE2b-256 | 031c125ccc4e2e1cb43d10243431795ddb9c8c5bb8a8866195df77eeed3ba9c8 |
File details
Details for the file exert-0.3.1-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
- Upload date:
- Size: 77.3 kB
- Tags: CPython 3.7m, manylinux: glibc 2.17+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | f3489a98ff822aa234251cdd521796af7885b2de679b1581fbe94aeb05df4822 |
|
MD5 | a4d8b4bc3cbd2771c5b4d6feac19dae7 |
|
BLAKE2b-256 | 5cfd34541dea31eb4acdd86554f4e9b84628b2b1936c1a021357c559ae45daee |
File details
Details for the file exert-0.3.1-cp37-cp37m-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
.
File metadata
- Download URL: exert-0.3.1-cp37-cp37m-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
- Upload date:
- Size: 77.6 kB
- Tags: CPython 3.7m, manylinux: glibc 2.17+ i686, manylinux: glibc 2.5+ i686
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 6c69cd783360824369e4256e0bf67040857e032b2814dafe7a673cec1fe41cd2 |
|
MD5 | c090de135f2538a8fda578542888016b |
|
BLAKE2b-256 | 1b8413087e48b59b2c0b6b35f9571599d759201985d376e5ded646c7ef44f1cd |
File details
Details for the file exert-0.3.1-cp37-cp37m-macosx_10_9_x86_64.whl
.
File metadata
- Download URL: exert-0.3.1-cp37-cp37m-macosx_10_9_x86_64.whl
- Upload date:
- Size: 47.5 kB
- Tags: CPython 3.7m, macOS 10.9+ x86-64
- Uploaded using Trusted Publishing? No
- Uploaded via: twine/4.0.0 CPython/3.9.12
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0c216800c6d78f5f77999c7614acc64cc9b8bae39eb1b9c1d2d0c55a92371d2e |
|
MD5 | 07e855cb56ad9692275b69b68ba1d493 |
|
BLAKE2b-256 | 842d99a27c8e285cf6c2f8afa3cea37aaa7c12474b717349b2b0b6e08fc10d17 |