Declarively apply converter functions to class attributes.
Project description
Exert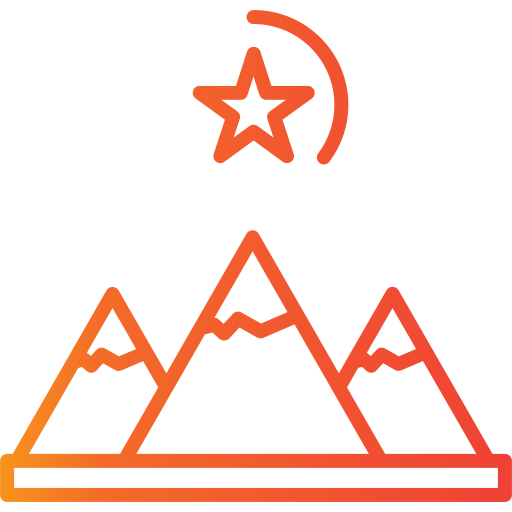
>> Declaratively apply converter functions to class attributes. <<
Installation
Install via pip:
pip install exert
Usage
Use this to declaratively apply arbitrary converter functions to the attributes of a class. For example:
from __future__ import annotations
from typing import Annotated
from exert import exert, Mark
@exert
class Foo:
a: Annotated[int, Mark(lambda x: x**2)]
b: Annotated[float, Mark(lambda x: x / 2)]
def __init__(self, a: int, b: float) -> None:
self.a = a
self.b = b
foo = Foo(2, 42.0)
print(foo.a) # prints 4
print(foo.b) # prints 21.0
Here, the lambda function tagged with Mark
is the converter.
Use with dataclasses
Dataclasses can also be used to avoid writing the initializer by hand. For example:
...
from dataclasses import dataclass
@exert
@datclasses
class Foo:
a: Annotated[int, Mark(lambda x: x**2)]
b: Annotated[float, Mark(lambda x: x / 2)]
foo = Foo(2, 42.0)
print(foo.a) # prints 4
print(foo.b) # prints 21.0
Apply multiple converters sequentially
Multiple converters are allowed. For example:
...
@exert
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x**2, lambda x: x**3)]
b: Annotated[float, Mark(lambda x: x / 2, lambda x: x / 3)]
foo = Foo(2, 42.0)
print(foo.a) # prints 64 [2**2=4, 4**3=64]
print(foo.b) # prints 7.0 [42.0/2=21.0, 21.0/3=7.0]
Here, the converters are applied sequentially. The result of the preceding converter is fed into the succeeding converter as input. You've to make sure that the number of the returned values of the preceding converter matches that of the succeeding converter.
Exclude annotated fields
If you don't wrap converters with Mark
, the corresponding field won't be transformed:
...
@exert
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x**2, lambda x: x**3)]
b: Annotated[float, lambda x: x / 2, lambda x: x / 3]
foo = Foo(2, 42.0)
print(foo.a) # prints 64 [2**2=4, 4**3=64]
print(foo.b) # prints 42.0 [This field was ignored]
Since the converters in field b
weren't tagged with Mark
, no conversion happened.
Apply common converters without repetition
Common converters can be applied to multiple fields without repetition:
...
@exert(converters=(lambda x: x**2,))
@dataclass
class Foo:
a: Annotated[int, None]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 4 [2**2=4]
print(foo.b) # prints 1764.0 [42.0**2=1764.0]
Apply common and marked converters together
You can apply a sequence of common converters and marked converters together. By default, the common converters are applied first and then the tagged converters are applied sequentially:
...
@exert(converters=(lambda x: x**2, lambda x: x**3))
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x / 100)]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 0.64 [2**2=4, 4**3=64, 64/100=0.64]
print(foo.b) # prints 5489031744.0 [42.0**2=1764, 1764**3=5489031744.0]
You can also, choose to apply the common converters after the tagged ones. For this,
you'll need to set the apply_last
parameter to True
:
...
@exert(
converters=(lambda x: x**2, lambda x: x**3),
apply_last=True,
)
@dataclass
class Foo:
a: Annotated[int, Mark(lambda x: x / 100)]
b: Annotated[float, None]
foo = Foo(2, 42.0)
print(foo.a) # prints 6.401e-11 [2/100=0.02, 0.02**2=0.004, 0.0004**3=6.401e-11]
print(foo.b) # prints 5489031744.0 [42.0**2=1764, 1764**3=5489031744.0]
Simple data validation
The snippet below ensures that the attributes of the class Foo
conforms to the constraints imposed by the converter functions.
from __future__ import annotations
import json
from dataclasses import dataclass
from functools import partial
from typing import Annotated, Sized
from exert import Mark, exert
def assert_len(x: Sized, length: int) -> Sized:
"""Assert that the incoming attribute has the expected length."""
assert len(x) == length
return x
def assert_json(x: dict) -> dict:
"""Assert that the incoming attribute is JSON serializable."""
try:
json.dumps(x)
except TypeError:
raise AssertionError(f"{x} is not JSON serializable")
return x
@exert
@dataclass
class Foo:
a: Annotated[tuple[int, ...], Mark(partial(assert_len, length=3))]
b: Annotated[dict, Mark(assert_json)]
foo = Foo(a=(1, 2, 3), b={"a": 1, "b": 2})
print(foo)
This will raise AssertionError
if the value of the attributes violate the constraints.
Project details
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distributions
Hashes for exert-0.3.3-cp311-cp311-musllinux_1_1_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | d90e71b06f211301cdf78581c98057533a57bfa3e1fd5b3f152c11d62a29a7e9 |
|
MD5 | 2a61164233a4c71d019b8dbc639f5f7f |
|
BLAKE2b-256 | b21a726b433aa7f4715922de60317ced76e39a9f38c0739222de83df45daa226 |
Hashes for exert-0.3.3-cp311-cp311-musllinux_1_1_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 25bd13186b0bc4ffe288f0e4da4d464f6c4af3021c0cae5b6e90519199176f08 |
|
MD5 | 6f3ec33fde3bf8d9256640e8a2bcfba7 |
|
BLAKE2b-256 | 9c353bf7407cecff2dd190101c71b43645ea7204a8a37e4ea9ffcffbca5b7487 |
Hashes for exert-0.3.3-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 3018d0e697d98f4c9d9fa3b46e0ec2d42f840cf0c0aea98787ea93ef7fabd720 |
|
MD5 | 1ca46d8d37f79ab18c43061840d9b184 |
|
BLAKE2b-256 | c1f0d9697143d7a5611f7efa64edb06ecbd3fdf4de411a232c9a49f4b651168e |
Hashes for exert-0.3.3-cp311-cp311-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0966647873cff13e04dd9d67b1b8ccd5dccd5e2cede58ad30f8e29367af96b1a |
|
MD5 | b00e40016a0cf325c6d55bd7a5ea3b2f |
|
BLAKE2b-256 | 9cab2aa42e0a8d3b44802ff93665b8ccb97a506ecbd3e9f97f3cc97e8e290100 |
Hashes for exert-0.3.3-cp311-cp311-macosx_10_9_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 7527fa30f4a178d6a1615939c9881095856960e699c99dd1a583cc56c173a7cd |
|
MD5 | 1f85746f70f25a4286b252d42a3fd923 |
|
BLAKE2b-256 | f8f7329323cb14dfb175cca06146b308810e5861e773b512571de37272056466 |
Hashes for exert-0.3.3-cp310-cp310-musllinux_1_1_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 3ba5cb2dcc06442cb54e26bd5d849512f479ed82af530fa9315880c5988baeb1 |
|
MD5 | 24f96e47a4dc7a2c79a277b984fbce0d |
|
BLAKE2b-256 | 63d417cb7e6a8604446b11255d832a4ac5d64303b19aee449a071eeb2e05b032 |
Hashes for exert-0.3.3-cp310-cp310-musllinux_1_1_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 2b53aae1c6f4412b92425f10c4ada314fd4247be7b0c807b9a0fe7412bc2fdad |
|
MD5 | af05e771355dfd9a88f8aff49c95a536 |
|
BLAKE2b-256 | ce6e76c67da8e53382b0b15ae3bcf48124c23fc7742d0efd2f70c68bb8665507 |
Hashes for exert-0.3.3-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 7616baad9167ab4c213e4f8d1800b6f67b6390f4d1b9b1bdb4c4920e4c770bc0 |
|
MD5 | 6304659d35e3e52c7714f985bf827f7a |
|
BLAKE2b-256 | 3ab9a6313a6cce9f1b61bcf20d0fc2b1ecf4fb4d798ea78529888dafb53429bd |
Hashes for exert-0.3.3-cp310-cp310-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | b8dba95e54c5aea67d0880c84c91756ffd5d12be7a7c9f36f042542044d08632 |
|
MD5 | a105f6871673b600c8bea5e99125e5f6 |
|
BLAKE2b-256 | a1bb5bed1a4c227090b108f3ffe355934737e8338e290276cf6c7d26e88eaa58 |
Hashes for exert-0.3.3-cp310-cp310-macosx_10_9_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | f19c8962d1db4eb6d254998cb9a6582dee6df43bedfc67d797ad2d2787debf20 |
|
MD5 | 4860edc2b942fd03bb3253bc05eef89c |
|
BLAKE2b-256 | 3da75178b60951c6a834afe66f77918dff72ab7fbb6b93a84cb7f6197273acd9 |
Hashes for exert-0.3.3-cp39-cp39-musllinux_1_1_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0c893155de45bdef8258d0076939f5cc6af51c6550df9b9e2d98a83e8a10a717 |
|
MD5 | cd782e1d02906bd62c778c330789947a |
|
BLAKE2b-256 | 809d7c08a50bad4675aa0848d629a252b715eee8e1527be67b28b9b3c95de581 |
Hashes for exert-0.3.3-cp39-cp39-musllinux_1_1_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | e9541555f2c20196456cc764fa7877a21beb30441306db3613784ed45d499b4f |
|
MD5 | b2c809fc37c12e7bfe8e7287164d1e86 |
|
BLAKE2b-256 | bf5403d7cb54aba4ed93929d59bdb90e841efe3f705c07432a2e03bdd85108a6 |
Hashes for exert-0.3.3-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 0a40ca9d432fb4faf17184fba53e69acb98bc788312975b77adedb5ae5052ce2 |
|
MD5 | 7c98e53b786863c00068ec9c1a6e8eaa |
|
BLAKE2b-256 | faf5ff90c243e00356bb92610b9d3833f3e72f30272e17d36a9efc9ea432d91a |
Hashes for exert-0.3.3-cp39-cp39-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | c22c806499096f745289c4097e5a6be86db722d1907ece1e2cca86800fc61de8 |
|
MD5 | 5a647c7c213e5ae237dbffeae4163674 |
|
BLAKE2b-256 | d1d9197e91b90a14e441d28ac3a948a0225b1c9b6529be8f12b9432f80af7c44 |
Hashes for exert-0.3.3-cp39-cp39-macosx_10_9_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 52fd6dc78c7ed9068dc8110f9a9c9443c629081a241df2048829666b6f492651 |
|
MD5 | 6780b660a37503344e6758759204f54b |
|
BLAKE2b-256 | a9b1d94106d28e5d70dfe8f68d37a1ca42f753c56b1dfd713ef870f0bcab4edb |
Hashes for exert-0.3.3-cp38-cp38-musllinux_1_1_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 43a23c00b11a5cc47a0c11598da634e28c2484e4f2d08042b2fd8dd2bba09f79 |
|
MD5 | 8abf749e71c9d32e0ec79fa1bfde2a93 |
|
BLAKE2b-256 | 6e9ea69991ff2883c92d72baf6bed09730d8a39c4b7604dd53da3381729dfd2a |
Hashes for exert-0.3.3-cp38-cp38-musllinux_1_1_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 82baee6d4495b7d733120e2ff155f84335941b5e22040e049d4eb45a41645730 |
|
MD5 | 7f358f0cd5b8262d1db44a6651019de8 |
|
BLAKE2b-256 | 6eb5daaa0eb4411d15ac4c2cc83c52ae3db7da85acce4c3ba1b64a92b9115d83 |
Hashes for exert-0.3.3-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | cba40c47ccce437cd749c48b662989518e9e5c5d87d10c1710a8897115cea6a4 |
|
MD5 | d9fc8db7c503119c41ac3ad56ce8729e |
|
BLAKE2b-256 | 4012a34081c86714d7da725f3effdd7564d0ab37c9e891761dc63b4376622860 |
Hashes for exert-0.3.3-cp38-cp38-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 659d92cde32125d7d2b8fd1867fb3c12e28107d0031b690022ae3e4947303d8d |
|
MD5 | 8c43fc03500e71f4a7b322d872d8f2e0 |
|
BLAKE2b-256 | 04c50d59f428b596334d741f0d030343ebcebd2bffc838aa41c4c72f9508f7ca |
Hashes for exert-0.3.3-cp38-cp38-macosx_10_9_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 5142e6a82064256c769755adb9a21ce3d3e4c5a293a6e6220317a84adf7c1043 |
|
MD5 | 83241add2ebf54973f9e8e0e522b6eb3 |
|
BLAKE2b-256 | 4c13b2fa4cb7de908fc7582d9038948dc8ce2d83cff8621cd6718d32e00979d1 |
Hashes for exert-0.3.3-cp37-cp37m-musllinux_1_1_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 29d3e31e903a83304315b64ecd07b71c80c96699616f5d83dc8229a7debcc093 |
|
MD5 | 540be76f350b24c07d59a2bfe920354f |
|
BLAKE2b-256 | 31aea69aa64a57c1f858fcf4ef0493fa89c205df9fd19e5d68539abf656bb09d |
Hashes for exert-0.3.3-cp37-cp37m-musllinux_1_1_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 22f0beea5de22a1d2a18c023ac61eb6edadc45679590c59f8917d17dfe6075ac |
|
MD5 | e3a7650708a0dbc4f7732e094f9c5276 |
|
BLAKE2b-256 | c17766f4ed39de0861e329b949d74e39fa1b2350d0f95d1687e43ee76261361b |
Hashes for exert-0.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | fee3ed2f0f917106f9faeb3d1f235312b90aa544b3f7324a52a73056f5bf8d96 |
|
MD5 | da5ed014f6abe17fc3a6cebf09db2c0b |
|
BLAKE2b-256 | fcdde104e566cadee614d2c561215585378ada8a4d84f693a965c4fe7a617f3d |
Hashes for exert-0.3.3-cp37-cp37m-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 85b3ed4ed2d4eeaab05bdb90cd80cf92fceb541a6200a631a5e5e5344b3b2328 |
|
MD5 | 5c011e2e443e04a8fd0627244a663eab |
|
BLAKE2b-256 | eee2141fe0f9530975299e0ad390c4559589135b9ec7dbc82b52026ff467f77c |
Hashes for exert-0.3.3-cp37-cp37m-macosx_10_9_x86_64.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 8843437d4b24b1536a804dd5ecaf96c971861de75f6fd2d272b05f7048f5681e |
|
MD5 | c0ffed38fe69b620851e8136c6016116 |
|
BLAKE2b-256 | 437050f8e91c8f8aacb7b92b35a109dd514b0550a37c1cf2e29a1761366a55d5 |