Easy to setup OAuth2 authentication with support for several auth providers.
Project description
fastapi-oauth2 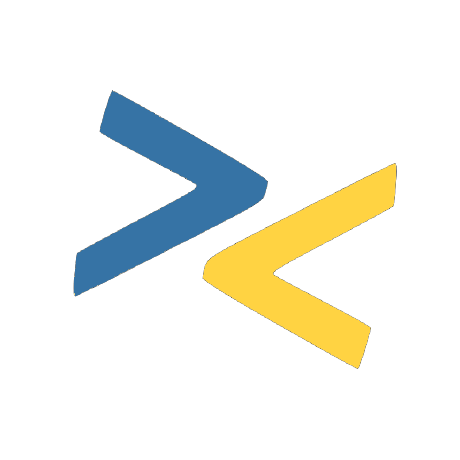
FastAPI OAuth2 is a middleware-based social authentication mechanism supporting several auth providers. It depends on the social-core authentication backends.
Features to be implemented
- Use multiple OAuth2 providers at the same time
- There need to be provided a way to configure the OAuth2 for multiple providers
- Provide
fastapi.security.*
implementations that use cookies - Token -> user data, user data -> token easy conversion
- Customizable OAuth2 routes
- Registration support
Installation
python -m pip install fastapi-oauth2
Configuration
Configuration requires you to provide the JWT requisites and define the clients of the particular providers. The
middleware configuration is declared with the OAuth2Config
and OAuth2Client
classes.
OAuth2Config
allow_http
- Allow insecure HTTP requests. Defaults toFalse
.jwt_secret
- The secret key used to sign the JWT. Defaults toNone
.jwt_expires
- The expiration time of the JWT in seconds. Defaults to900
.jwt_algorithm
- The algorithm used to sign the JWT. Defaults toHS256
.clients
- The list of the OAuth2 clients. Defaults to[]
.
OAuth2Client
backend
- The social-core authentication backend classname.client_id
- The OAuth2 client ID for the particular provider.client_secret
- The OAuth2 client secret for the particular provider.redirect_uri
- The OAuth2 redirect URI to redirect to after success. Defaults to the base URL.scope
- The OAuth2 scope for the particular provider. Defaults to[]
.
It is also important to mention that for the configured clients of the auth providers, the authorization URLs are
accessible by the /oauth2/{provider}/auth
path where the provider
variable represents the exact value of the auth
provider backend name
attribute.
from fastapi_oauth2.client import OAuth2Client
from fastapi_oauth2.config import OAuth2Config
from social_core.backends.github import GithubOAuth2
oauth2_config = OAuth2Config(
allow_http=False,
jwt_secret=os.getenv("JWT_SECRET"),
jwt_expires=os.getenv("JWT_EXPIRES"),
jwt_algorithm=os.getenv("JWT_ALGORITHM"),
clients=[
OAuth2Client(
backend=GithubOAuth2,
client_id=os.getenv("OAUTH2_CLIENT_ID"),
client_secret=os.getenv("OAUTH2_CLIENT_SECRET"),
redirect_uri="https://pysnippet.org/",
scope=["user:email"],
),
]
)
Integration
To integrate the package into your FastAPI application, you need to add the OAuth2Middleware
with particular configs
in the above-represented format and include the router to the main router of the application.
from fastapi import FastAPI
from fastapi_oauth2.middleware import OAuth2Middleware
from fastapi_oauth2.router import router as oauth2_router
app = FastAPI()
app.include_router(oauth2_router)
app.add_middleware(OAuth2Middleware, config=oauth2_config)
After adding the middleware, the user
attribute will be available in the request context. It will contain the user
data provided by the OAuth2 provider.
{% if request.user.is_authenticated %}
<a href="/oauth2/logout">Sign out</a>
{% else %}
<a href="/oauth2/github/auth">Sign in</a>
{% endif %}
Contribute
Any contribution is welcome. If you have any ideas or suggestions, feel free to open an issue or a pull request. And don't forget to add tests for your changes.
License
Copyright (C) 2023 Artyom Vancyan. MIT
Project details
Release history Release notifications | RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distribution
Hashes for fastapi_oauth2-1.0.0a0-py3-none-any.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 8d03ddf8420a7a12dd2c97a5d06a859949dbac98fd9e832ae924d8ea7649eb48 |
|
MD5 | ff2d0095d564b3c07c71c7dbec8a35c8 |
|
BLAKE2b-256 | c92f04d300e16089d10e12cda023d34b6688bd7090ec2cd377cd6ded77dce8f2 |