🐍 Soothing pastel theme for Python.
Project description
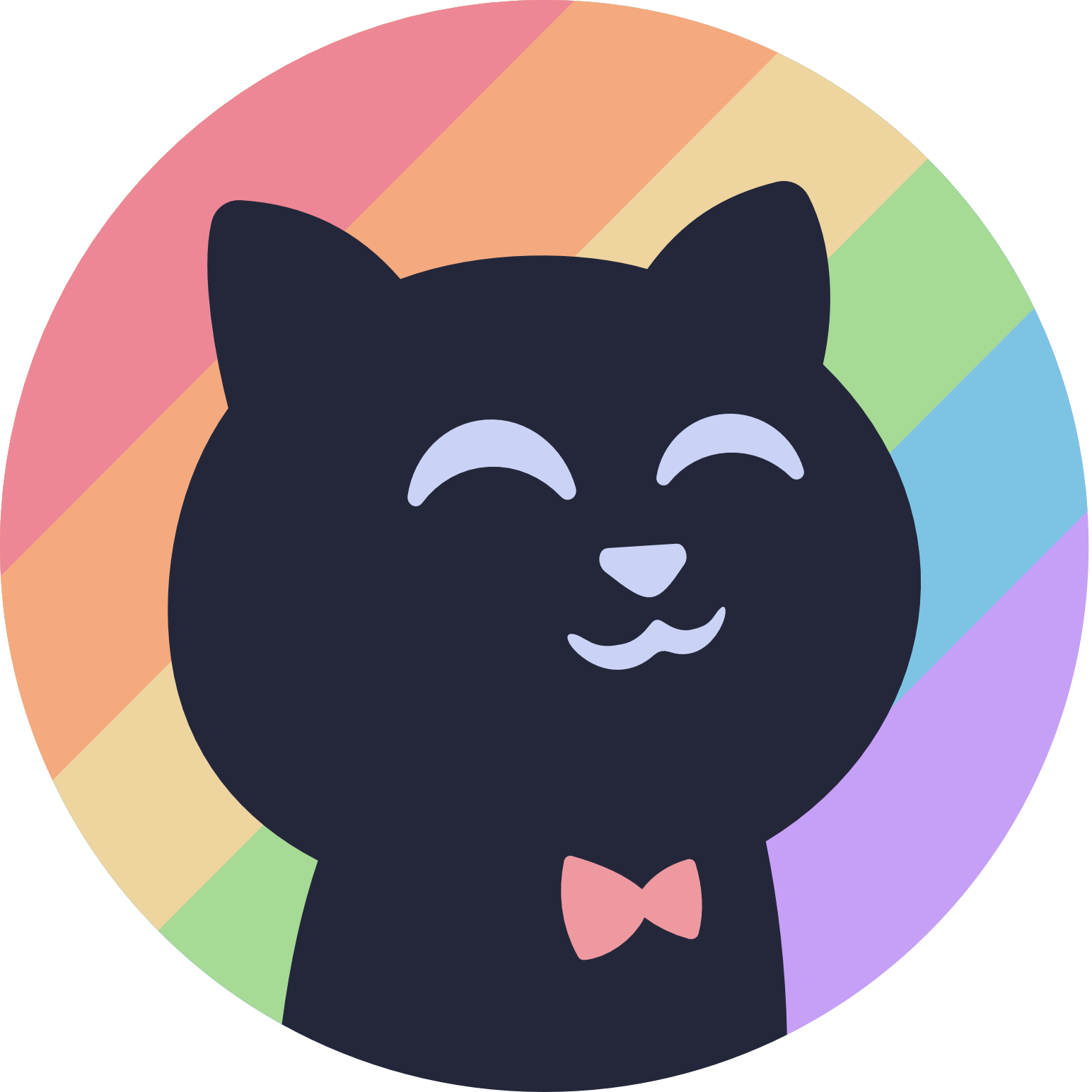
Catppuccin for Python
Installation
Install with pip
or your preferred dependency management tool.
pip install catppuccin
Usage
Get access to the palette with the catppuccin.PALETTE
constant:
from catppuccin import PALETTE
PALETTE.latte.colors.mauve.hex
# '#8839ef'
PALETTE.mocha.colors.teal.rgb
# RGB(r=148, g=226, b=213)
The Palette
data structure matches the palette JSON.
Iteration
Both Palette
and FlavorColors
can be iterated to yield flavors and colors respectively:
for flavor in PALETTE:
print(flavor.name)
# Latte
# Frappé
# Macchiato
# Mocha
for color in PALETTE.latte.colors:
print(f"{color.name}: {color.hex}")
# Rosewater: #f2d5cf
# Flamingo: #eebebe
# Pink: #f4b8e4
# ...
# Base: #303446
# Mantle: #292c3c
# Crust: #232634
dataclasses
Palette
, Flavor
, Color
et cetera are all dataclasses
,
so you can also inspect and iterate their fields using methods from the dataclass module.
For example, to list all color names and their hex codes:
from dataclasses import fields
from catppuccin import PALETTE
flavor = PALETTE.frappe
for field in fields(flavor.colors):
color = getattr(flavor.colors, field.name)
print(f"{field.name}: {color.hex}")
# rosewater: #f2d5cf
# flamingo: #eebebe
# pink: #f4b8e4
# ...
# base: #303446
# mantle: #292c3c
# crust: #232634
Pygments Styles
This package provides a Pygments style for each of the four Catppuccin flavors.
Install Catppuccin with the pygments
feature to include the relevant dependencies:
pip install catppuccin[pygments]
The styles are registered as importlib entrypoints, which allows Pygments to find them by name:
from pygments.styles import get_style_by_name
get_style_by_name("catppuccin-frappe")
# catppuccin.extras.pygments.FrappeStyle
The following style names are available:
catppuccin-latte
catppuccin-frappe
catppuccin-macchiato
catppuccin-mocha
They can also be accessed by directly importing them:
from catppuccin.extras.pygments import MacchiatoStyle
IPython
A minimal configuration:
c.TerminalInteractiveShell.true_color = True
c.TerminalInteractiveShell.highlighting_style = "catppuccin-mocha"
Putting this into your IPython configuration
and ensuring catppuccin[pygments]
is installed in the same environment will
give you Catppuccin Mocha syntax highlighting in the REPL. See here
for an example of a more complete configuration.
Matplotlib
The library tries to register styles and colormaps if matplotlib
is installed.
See the examples below for some use cases:
- Load a style, using
mpl.style.use
import catppuccin import matplotlib as mpl import matplotlib.pyplot as plt mpl.style.use(catppuccin.PALETTE.mocha.identifier) plt.plot([0,1,2,3], [1,2,3,4]) plt.show()
- Mix it with different stylesheets!
import catppuccin import matplotlib as mpl import matplotlib.pyplot as plt mpl.style.use(["ggplot", catppuccin.PALETTE.mocha.identifier]) plt.plot([0,1,2,3], [1,2,3,4]) plt.show()
- Load individual colors
import matplotlib.pyplot as plt import catppuccin from catppuccin.extras.matplotlib import load_color color = load_color(catppuccin.PALETTE.latte.identifier, "peach") plt.plot([0,1,2,3], [1,2,3,4], color=color) plt.show()
- Define custom colormaps
import matplotlib.pyplot as plt import numpy as np import catppuccin from catppuccin.extras.matplotlib import get_colormap_from_list cmap = get_colormap_from_list( catppuccin.PALETTE.frappe.identifier, ["red", "peach", "yellow", "green"], ) rng = np.random.default_rng() data = rng.integers(2, size=(30, 30)) plt.imshow(data, cmap=cmap) plt.show()
Contribution
If you are looking to contribute, please read through our CONTRIBUTING.md first!
Development
This project is maintained with Poetry. If you don't have Poetry yet, you can install it using the installation instructions.
Install the project's dependencies including extras:
poetry install --all-extras
Codegen
catppuccin/palette.py
is generated by a build script based on the contents of palette.json
.
To update after downloading a new palette JSON file:
poetry run python build.py
Formatting this file is done manually as with any other file, see Code Standards
below.
Code Standards
Before committing changes, it is recommended to run the following tools to ensure consistency in the codebase.
ruff format
ruff check
mypy .
pytest --cov catppuccin
These tools are all installed as part of the dev
dependency group with
Poetry. You can use poetry shell
to automatically put these tools in your
path.
💝 Thanks to
Copyright © 2022-present Catppuccin Org
Project details
Release history Release notifications | RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distribution
File details
Details for the file catppuccin-2.3.2.tar.gz
.
File metadata
- Download URL: catppuccin-2.3.2.tar.gz
- Upload date:
- Size: 17.0 kB
- Tags: Source
- Uploaded using Trusted Publishing? No
- Uploaded via: poetry/1.8.3 CPython/3.12.5 Linux/6.5.0-1025-azure
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 9a2a470724eb231b56895abc4239844168126e90e808c16d9bba8a1d036d7a44 |
|
MD5 | e07161eaa86aee03bcdb3cce1aa9781e |
|
BLAKE2b-256 | 6dbe3afbb5cb8e4422ed66365cc3f1504bdff7cadce09881d99b65e88397059a |
File details
Details for the file catppuccin-2.3.2-py3-none-any.whl
.
File metadata
- Download URL: catppuccin-2.3.2-py3-none-any.whl
- Upload date:
- Size: 18.6 kB
- Tags: Python 3
- Uploaded using Trusted Publishing? No
- Uploaded via: poetry/1.8.3 CPython/3.12.5 Linux/6.5.0-1025-azure
File hashes
Algorithm | Hash digest | |
---|---|---|
SHA256 | 7ee89da15e1e7fca380602734ca105c3642e10aee4e90899335974f797c635e2 |
|
MD5 | d25a500527c741a5fb2a0b1d5fcfd3cd |
|
BLAKE2b-256 | 4cfd70b48870afa2315057970f6a8a268f0f4064ed344e88acfbcdacaf6e5118 |