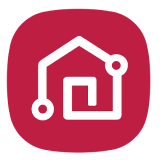
Project Description
The thinqconnect provides a robust interface for interacting with the LG ThinQ Connect Open API.
This SDK is designed to facilitate seamless integration with a range of LGE appliances, bases on LG ThinQ Connect.
Key Features
- Profile Retrieval: Access detailed profiles of 27 different home appliances.
- Device Management: Query and retrieve lists of connected devices and their statuses.
- Device Control: Execute commands to control your appliances directly through the API.
- Event Handling: Utilize AWS IoT Core for MQTT connections to receive device events and push notifications via callbacks.
This SDK is an essential tool for developers looking to integrate ThinQ Connect capabilities into their applications, ensuring efficient and reliable smart home management.
Installation and usage
Installation
pip install thinqconnect
Usage
Obtaining and Using a Personal Access Token
To use the ThinQ Connect Python SDK, you need to obtain a Personal Access Token from the LG ThinQ Developer Site.
Follow the steps below to get your token and configure your environment.
Steps to Obtain a Personal Access Token
- Sign Up or Log In:
- Navigate to Cloud Developer:
- After logging in, go to the Cloud Developer section.
- Navigate to Docs.
- Locate ThinQ Connect:
- Within the docs, find and select ThinQ Connect.
- Generate Personal Access Token:
- Under the ThinQ Connect section, locate PAT (Personal Access Token).
- If you don’t have an account, sign up for one. If you already have an account, log in using your LG ThinQ Account.
- Follow the instructions provided to generate and copy your Personal Access Token.
After obtaining your Personal Access Token, you need to configure your environment to use it with the SDK.
Client ID Requirements
Each client device must use a unique Client ID. This Client ID should be a randomly generated value, and using a uuid4 format is recommended.
Be cautious with excessive client creation, as it may lead to your API calls being blocked.
import uuid
client_id = str(uuid.uuid4())
Country Codes
When initializing the SDK, you will also need to provide a country code.
Refer to the table below for the appropriate country code to use:
Country |
Code |
Country |
Code |
Country |
Code |
AE |
United Arab Emirates |
GD |
Grenada |
NG |
Nigeria |
AF |
Afghanistan |
GE |
Georgia |
NI |
Nicaragua |
AG |
Antigua and Barbuda |
GH |
Gana |
NL |
Netherlands |
AL |
Albania |
GM |
Gambia |
NO |
Norway |
AM |
Armenia |
GN |
Guinea |
NP |
Nepal |
AO |
Angola |
GQ |
Equatorial Guinea |
NZ |
New Zealand |
AR |
Argentina |
GR |
Greece |
OM |
Oman |
AT |
Austria |
GT |
Guatemala |
PA |
Panama |
AU |
Australia |
GY |
Guyana |
PE |
Peru |
AW |
Aruba |
HK |
Hong Kong |
PH |
Philippines |
AZ |
Azerbaijan |
HN |
Honduras |
PK |
Pakistan |
BA |
Bosnia and Herzegovina |
HR |
Croatia |
PL |
Poland |
BB |
Barbados |
HT |
Haiti |
PR |
Puerto Rico |
BD |
Bangladesh |
HU |
Hungary |
PS |
Occupied Palestinian Territory |
BE |
Belgium |
ID |
Indonesia |
PT |
Portugal |
BF |
Burkina Faso |
IE |
Ireland |
PY |
Paraguay |
BG |
Bulgaria |
IL |
Israel |
QA |
Qatar |
BH |
Bahrain |
IN |
India |
RO |
Romania |
BJ |
Benin |
IQ |
Iraq |
RS |
Serbia |
BO |
Bolivia |
IR |
Iran |
RU |
Russian Federation |
BR |
Brazil |
IS |
Iceland |
RW |
Rwanda |
BS |
Bahamas |
IT |
Italy |
SA |
Saudi Arabia |
BY |
Belarus |
JM |
Jamaica |
SD |
Sudan |
BZ |
Belize |
JO |
Jordan |
SE |
Sweden |
CA |
Canada |
JP |
Japan |
SG |
Singapore |
CD |
Democratic Republic of the Congo |
KE |
Kenya |
SI |
Slovenia |
CF |
Central African Republic |
KG |
Kyrgyzstan |
SK |
Slovakia |
CG |
Republic of the Congo |
KH |
Cambodia |
SL |
Sierra Leone |
CH |
Switzerland |
KN |
Saint Kitts and Nevis |
SN |
Senegal |
CI |
Republic of Ivory Coast |
KR |
Korea |
SO |
Somalia |
CL |
Chile |
KW |
Kuwait |
SR |
Suriname |
CM |
Cameroon |
KZ |
Kazakhstan |
ST |
Sao Tome and Principe |
CN |
China |
LA |
Laos |
SV |
El Salvador |
CO |
Colombia |
LB |
Lebanon |
SY |
Syrian Arab Republic |
CR |
Costa Rica |
LC |
Saint Lucia |
TD |
Chad |
CU |
Cuba |
LK |
Sri Lanka |
TG |
Togo |
CV |
Cape Verde |
LR |
Liberia |
TH |
Thailand |
CY |
Cyprus |
LT |
Lithuania |
TN |
Tunisia |
CZ |
Czech Republic |
LU |
Luxembourg |
TR |
Turkey |
DE |
Germany |
LV |
Latvia |
TT |
Trinidad and Tobago |
DJ |
Djibouti |
LY |
Libyan Arab Jamahiriya |
TW |
Taiwan |
DK |
Denmark |
MA |
Morocco |
TZ |
United Republic of Tanzania |
DM |
Dominica |
MD |
Republic of Moldova |
UA |
Ukraine |
DO |
Dominican Republic |
ME |
Montenegro |
UG |
Uganda |
DZ |
Algeria |
MK |
Macedonia |
US |
USA |
EC |
Ecuador |
ML |
Mali |
UY |
Uruguay |
EE |
Estonia |
MM |
Myanmar |
UZ |
Uzbekistan |
EG |
Egypt |
MR |
Mauritania |
VC |
Saint Vincent and the Grenadines |
ES |
Spain |
MT |
Malta |
VE |
Venezuela |
ET |
Ethiopia |
MU |
Mauritius |
VN |
Vietnam |
FI |
Finland |
MW |
Malawi |
XK |
Kosovo |
FR |
France |
MX |
Mexico |
YE |
Yemen |
GA |
Gabon |
MY |
Malaysia |
ZA |
South Africa |
GB |
United Kingdom |
NE |
Niger |
ZM |
Zambia |
Simple Test
import asyncio
from aiohttp import ClientSession
from thinqconnect.thinq_api import ThinQApi
async def test_devices_list():
async with ClientSession() as session:
thinq_api = ThinQApi(session=session, access_token='your_personal_access_token', country_code='your_contry_code', client_id='your_client_id')
response = await thinq_api.async_get_device_list()
print("device_list : %s", response.body)
asyncio.run(test_devices_list())
Ensure that you keep your Personal Access Token and Client ID secure and do not expose them in your source code or public repositories.
License
Apache License
Available Device Types and Properties
For detailed information on Device Properties, please refer to the following page: LG ThinQ Connect - Device Profile
DEVICE_AIR_CONDITIONER
Main
|
resources |
properties |
1 |
air_con_job_mode |
current_job_mode |
2 |
operation |
air_con_operation_mode |
3 |
operation |
air_clean_operation_mode |
4 |
temperature |
current_temperature |
5 |
temperature |
target_temperature |
6 |
temperature |
heat_target_temperature |
7 |
temperature |
cool_target_temperature |
8 |
temperature |
temperature_unit |
9 |
two_set_temperature |
two_set_current_temperature |
10 |
two_set_temperature |
two_set_heat_target_temperature |
11 |
two_set_temperature |
two_set_cool_target_temperature |
12 |
two_set_temperature |
two_set_temperature_unit |
13 |
timer |
relative_hour_to_start |
14 |
timer |
relative_minute_to_start |
15 |
timer |
relative_hour_to_stop |
16 |
timer |
relative_minute_to_stop |
17 |
timer |
absolute_hour_to_start |
18 |
timer |
absolute_minute_to_start |
19 |
timer |
absolute_hour_to_stop |
20 |
timer |
absolute_minute_to_stop |
21 |
sleep_timer |
sleep_timer_relative_hour_to_stop |
22 |
sleep_timer |
sleep_timer_relative_minute_to_stop |
23 |
power_save |
power_save_enabled |
24 |
air_flow |
wind_strength |
25 |
air_flow |
wind_step |
26 |
air_quality_sensor |
pm1 |
27 |
air_quality_sensor |
pm2 |
28 |
air_quality_sensor |
pm10 |
29 |
air_quality_sensor |
odor |
30 |
air_quality_sensor |
humidity |
31 |
air_quality_sensor |
total_pollution |
32 |
air_quality_sensor |
monitoring_enabled |
33 |
filter_info |
used_time |
34 |
filter_info |
filter_lifetime |
DEVICE_AIR_PURIFIER
Main
|
resources |
properties |
1 |
air_purifier_job_mode |
current_job_mode |
2 |
air_purifier_job_mode |
personalization_mode |
3 |
operation |
air_purifier_operation_mode |
4 |
timer |
absolute_hour_to_start |
5 |
timer |
absolute_minute_to_start |
6 |
timer |
absolute_hour_to_stop |
7 |
timer |
absolute_minute_to_stop |
8 |
air_flow |
wind_strength |
9 |
air_quality_sensor |
monitoring_enabled |
10 |
air_quality_sensor |
pm1 |
11 |
air_quality_sensor |
pm2 |
12 |
air_quality_sensor |
pm10 |
13 |
air_quality_sensor |
odor |
14 |
air_quality_sensor |
humidity |
15 |
air_quality_sensor |
total_pollution |
DEVICE_AIR_PURIFIER_FAN
Main
|
resources |
properties |
1 |
air_fan_job_mode |
current_job_mode |
2 |
operation |
air_fan_operation_mode |
3 |
timer |
absolute_hour_to_start |
4 |
timer |
absolute_minute_to_start |
5 |
timer |
absolute_hour_to_stop |
6 |
timer |
absolute_minute_to_stop |
7 |
sleep_timer |
sleep_timer_relative_hour_to_stop |
8 |
sleep_timer |
sleep_timer_relative_minute_to_stop |
9 |
air_flow |
warm_mode |
10 |
air_flow |
wind_temperature |
11 |
air_flow |
wind_strength |
12 |
air_flow |
wind_angle |
13 |
air_quality_sensor |
monitoring_enabled |
14 |
air_quality_sensor |
pm1 |
15 |
air_quality_sensor |
pm2 |
16 |
air_quality_sensor |
pm10 |
17 |
air_quality_sensor |
humidity |
18 |
air_quality_sensor |
temperature |
19 |
air_quality_sensor |
odor |
20 |
air_quality_sensor |
total_pollution |
21 |
display |
display_light |
22 |
misc |
uv_nano |
DEVICE_CEILING_FAN
Main
|
resources |
properties |
1 |
air_flow |
wind_strength |
2 |
operation |
ceiling_fan_operation_mode |
DEVICE_COOKTOP
Main
|
resources |
properties |
1 |
operation |
operation_mode |
Sub
|
resources |
properties |
1 |
cooking_zone |
current_state |
2 |
power |
power_level |
3 |
remote_control_enable |
remote_control_enabled |
4 |
timer |
remain_hour |
5 |
timer |
remain_minute |
DEVICE_DEHUMIDIFIER
Main
|
resources |
properties |
1 |
operation |
dehumidifier_operation_mode |
2 |
dehumidifier_job_mode |
current_job_mode |
3 |
humidity |
current_humidity |
4 |
air_flow |
wind_strength |
DEVICE_DISH_WASHER
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
dish_washing_status |
rinse_refill |
3 |
preference |
rinse_level |
4 |
preference |
softening_level |
5 |
preference |
machine_clean_reminder |
6 |
preference |
signal_level |
7 |
preference |
clean_light_reminder |
8 |
door_status |
door_state |
9 |
operation |
dish_washer_operation_mode |
10 |
remote_control_enable |
remote_control_enabled |
11 |
timer |
relative_hour_to_start |
12 |
timer |
relative_minute_to_start |
13 |
timer |
remain_hour |
14 |
timer |
remain_minute |
15 |
timer |
total_hour |
16 |
timer |
total_minute |
17 |
dish_washing_course |
current_dish_washing_course |
DEVICE_DRYER
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
operation |
dryer_operation_mode |
3 |
remote_control_enable |
remote_control_enabled |
4 |
timer |
remain_hour |
5 |
timer |
remain_minute |
6 |
timer |
total_hour |
7 |
timer |
total_minute |
8 |
timer |
relative_hour_to_stop |
9 |
timer |
relative_minute_to_stop |
10 |
timer |
relative_hour_to_start |
11 |
timer |
relative_minute_to_start |
DEVICE_HOME_BREW
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
recipe |
beer_remain |
3 |
recipe |
flavor_info |
4 |
recipe |
hop_oil_info |
5 |
recipe |
wort_info |
6 |
recipe |
yeast_info |
7 |
recipe |
recipe_name |
8 |
timer |
elapsed_day_state |
9 |
timer |
elapsed_day_total |
DEVICE_HOOD
Main
|
resources |
properties |
1 |
ventilation |
fan_speed |
2 |
lamp |
lamp_brightness |
3 |
operation |
hood_operation_mode |
4 |
timer |
remain_minute |
5 |
timer |
remain_second |
DEVICE_HUMIDIFIER
Main
|
resources |
properties |
1 |
humidifier_job_mode |
current_job_mode |
2 |
operation |
humidifier_operation_mode |
3 |
operation |
auto_mode |
4 |
operation |
sleep_mode |
5 |
operation |
hygiene_dry_mode |
6 |
timer |
absolute_hour_to_start |
7 |
timer |
absolute_hour_to_stop |
8 |
timer |
absolute_minute_to_start |
9 |
timer |
absolute_minute_to_stop |
10 |
sleep_timer |
sleep_timer_relative_hour_to_stop |
11 |
sleep_timer |
sleep_timer_relative_minute_to_stop |
12 |
humidity |
target_humidity |
13 |
humidity |
warm_mode |
14 |
air_flow |
wind_strength |
15 |
air_quality_sensor |
monitoring_enabled |
16 |
air_quality_sensor |
total_pollution |
17 |
air_quality_sensor |
pm1 |
18 |
air_quality_sensor |
pm2 |
19 |
air_quality_sensor |
pm10 |
20 |
air_quality_sensor |
humidity |
21 |
air_quality_sensor |
temperature |
22 |
display |
display_light |
23 |
mood_lamp |
mood_lamp_state |
DEVICE_KIMCHI_REFRIGERATOR
Main
|
resources |
properties |
1 |
refrigeration |
one_touch_filter |
2 |
refrigeration |
fresh_air_filter |
Sub
|
resources |
properties |
1 |
temperature |
target_temperature |
DEVICE_MICROWAVE_OVEN
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
timer |
remain_minute |
3 |
timer |
remain_second |
4 |
ventilation |
fan_speed |
5 |
lamp |
lamp_brightness |
DEVICE_OVEN
Main
|
resources |
properties |
1 |
info |
oven_type |
Sub
|
resources |
properties |
1 |
run_state |
current_state |
2 |
operation |
oven_operation_mode |
3 |
cook |
cook_mode |
4 |
remote_control_enable |
remote_control_enabled |
5 |
temperature |
target_temperature_c |
6 |
temperature |
target_temperature_f |
7 |
timer |
remain_hour |
8 |
timer |
remain_minute |
9 |
timer |
remain_second |
10 |
timer |
target_hour |
11 |
timer |
target_minute |
12 |
timer |
target_second |
13 |
timer |
timer_hour |
14 |
timer |
timer_minute |
15 |
timer |
timer_second |
DEVICE_PLANT_CULTIVATOR
Main - Empty
Sub
|
resources |
properties |
1 |
run_state |
current_state |
2 |
run_state |
growth_mode |
3 |
run_state |
wind_volume |
4 |
light |
brightness |
5 |
light |
duration |
6 |
light |
start_hour |
7 |
light |
start_minute |
8 |
light |
end_hour |
9 |
light |
end_minute |
10 |
temperature |
day_target_temperature |
11 |
temperature |
night_target_temperature |
DEVICE_REFRIGERATOR
Main
|
resources |
properties |
1 |
power_save |
power_save_enabled |
2 |
eco_friendly |
eco_friendly_mode |
3 |
sabbath |
sabbath_mode |
4 |
refrigeration |
rapid_freeze |
5 |
refrigeration |
express_mode |
6 |
refrigeration |
fresh_air_filter |
7 |
water_filter_info |
used_time |
8 |
water_filter_info |
water_filter_info_unit |
Sub
|
resources |
properties |
1 |
door_status |
door_state |
2 |
temperature |
location_name |
3 |
temperature |
target_temperature |
4 |
temperature |
temperature_unit |
DEVICE_ROBOT_CLEANER
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
robot_cleaner_job_mode |
current_job_mode |
3 |
operation |
clean_operation_mode |
4 |
battery |
battery_level |
5 |
battery |
battery_percent |
6 |
timer |
absolute_hour_to_start |
7 |
timer |
absolute_minute_to_start |
8 |
timer |
running_hour |
9 |
rtimer |
unning_minute |
DEVICE_STICK_CLEANER
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
stick_cleaner_job_mode |
current_job_mode |
3 |
battery |
battery_level |
DEVICE_STYLER
Main
|
resources |
properties |
1 |
run_state |
current_state |
2 |
operation |
styler_operation_mode |
3 |
remote_control_enable |
remote_control_enabled |
4 |
timer |
relative_hour_to_stop |
5 |
timer |
relative_minute_to_stop |
6 |
timer |
remain_hour |
7 |
timer |
remain_minute |
8 |
timer |
total_hour |
9 |
timer |
total_minute |
DEVICE_SYSTEM_BOILER
Main
|
resources |
properties |
1 |
boiler_job_mode |
current_job_mode |
2 |
operation |
boiler_operation_mode |
3 |
operation |
hot_water_mode |
4 |
temperature |
current_temperature |
5 |
temperature |
target_temperature |
6 |
temperature |
heat_target_temperature |
7 |
temperature |
cool_target_temperature |
8 |
temperature |
heat_max_temperature |
9 |
temperature |
heat_min_temperature |
10 |
temperature |
cool_max_temperature |
11 |
temperature |
cool_min_temperature |
12 |
temperature |
temperature_unit |
DEVICE_WASHER
Main - Empty
Sub
|
resources |
properties |
1 |
run_state |
current_state |
2 |
operation |
washer_operation_mode |
3 |
remote_control_enable |
remote_control_enabled |
4 |
timer |
remain_hour |
5 |
timer |
remain_minute |
6 |
timer |
total_hour |
7 |
timer |
total_minute |
8 |
timer |
relative_hour_to_stop |
9 |
timer |
relative_minute_to_stop |
10 |
timer |
relative_hour_to_start |
11 |
timer |
relative_minute_to_start |
12 |
detergent |
detergent_setting |
DEVICE_WATER_HEATER
Main
|
resources |
properties |
1 |
water_heater_job_mode |
current_job_mode |
2 |
operation |
water_heater_operation_mode |
3 |
temperature |
current_temperature |
4 |
temperature |
target_temperature |
DEVICE_WATER_PURIFIER
Main
|
resources |
properties |
1 |
run_state |
cock_state |
2 |
run_state |
sterilizing_state |
3 |
water_info |
water_type |
DEVICE_WINE_CELLAR
Main
|
resources |
properties |
1 |
operation |
light_brightness |
2 |
operation |
optimal_humidity |
3 |
operation |
sabbath_mode |
4 |
operation |
light_status |
Sub
|
resources |
properties |
1 |
temperature |
location_name |
2 |
temperature |
target_temperature |
3 |
temperature |
temperature_unit |