This package will help you to add authentication system and modern UI for your desktop based Python apps in a couple of seconds.
Project description
Firebase Authentication UI in Python Using Tkinter
This package will help you to add modern authentication UI in your desktop based Python apps by writing just 1 line. Just add this repository in the root folder of your project and write couple of lines to use Firebase authentication system in your python apps. Screenshots of the UI.
Please read the full documentation to implement it in your project. It is very simple!
Documentation
🐍 Just 1 line of python code to implement authentication and modern UI in Python
Install this package, get your Firebase configuration file and just write 1 line code. A complete example is given at the end of this documentation.
Simplest Firebase Authentication and Modern UI in Python using Tkinter!
First install the package
pip install firebase-auth-ui
Then import the package
from firebase_auth_ui import draw_ui
Now get your Firebase config values from your Firebase console and write 1 line python code
# Set your Firebase config values
firebase_config = {
"you will find it in your firebase console": "I have also added documentation about it"
}
# Run the authentication method
# Here my_app will be a function which contain your codes, basically you will replace the my_app
# with your main calling function which run/call your app
draw_ui.run_authentication(firebase_config, my_app)
# draw_ui.run_authentication() takes to arguments
# firebase_config_values and call_app
Create a Firebase Project and Get Firebase Config Values
- Go to https://console.firebase.google.com/
- Click "Add project"
- Give your project name (Any name you want) and click Continue
- Keep Google Analytics as same as it (Default is Enabled) given and click Continue
- Select "Default Account for Firebase" and click Create project
- Wait for a couple of seconds to get your project ready and when it is ready click Continue
- A dashboard of Project Overview will be opened and you will see iOS+, Android and Web
- Click on the Web which icon is </>
- Give an app name and don't select "Also set up Firebase Hosting for this app. Learn more" then click Register app
- Now you will see Add Firebase SDK
- Look throgh the SDK codes that is generated by Firebase
- You will see a const variable firebaseConfig which is your configuration values to use Firebase Authentication
- Copy the entire firebaseConfig values
-
apiKey: "XXxxXxX00XXxxx-xxxxX0XXXx0XxxxxxXXXXxxx", authDomain: "xxx-xx-xxxxx.xxxxxxxxxxx.com", projectId: "xxx-xx-xxxxx", storageBucket: "xxx-xx-xxxxx.xxxxxxxx.com", messagingSenderId: "xxxxxxxxxxxx", appId: "x:xxxxxxxxxxxx:web:xxxxxxxxxxxxxxxxxxxxxx", measurementId: "G-XXXXXXXXXX"}
- Click Continue to console
- Open your python script and create a dictionary variable firebase_config and paste the values like the given format
-
firebase_config={"apiKey": "XXxxXxX00XXxxx-xxxxX0XXXx0XxxxxxXXXXxxx", "authDomain": "xxx-xx-xxxxx.xxxxxxxxxxx.com", "projectId": "xxx-xx-xxxxx", "storageBucket": "xxx-xx-xxxxx.xxxxxxxx.com", "messagingSenderId": "xxxxxxxxxxxx", "appId": "x:xxxxxxxxxxxx:web:xxxxxxxxxxxxxxxxxxxxxx", "measurementId": "G-XXXXXXXXXX", "databaseURL": "" } # Copy this format exactly and DON"T FORGET TO KEEP "databaseURL":"", an empty string # Keep databaseURL value an empty string # See I have put the keys inside "" # You need to put all the keys inside "" as python only accepts this format in its dictionary data types
- Don't forget to add "databaseURL":"" like this, keep the value of "databaseURL" = empty string
- Go to your Firebase Project Overview/Dashboard and last one thing need to configure to use Firebase Authentication
- Click Build from left side menu
- Click Authentication
- Click Get Started
- From Sign-in method select Email/Password and Enable it
- Click Save
That's all from Firebase console.
An example of complete implementation of the package in your project
from firebase_auth_ui import draw_ui
# Your main app calling function
# For example, here my_app() is the final function which will be called to run the entire app
def my_app():
print(f"User Authentication Successful. The app is opening!")
# Set Firebase config
firebase_config={"apiKey": "XXxxXxX00XXxxx-xxxxX0XXXx0XxxxxxXXXXxxx",
"authDomain": "xxx-xx-xxxxx.xxxxxxxxxxx.com",
"projectId": "xxx-xx-xxxxx",
"storageBucket": "xxx-xx-xxxxx.xxxxxxxx.com",
"messagingSenderId": "xxxxxxxxxxxx",
"appId": "x:xxxxxxxxxxxx:web:xxxxxxxxxxxxxxxxxxxxxx",
"measurementId": "G-XXXXXXXXXX"}
draw_ui.run_authentication(firebase_config, my_app)
bada bim bada boom! 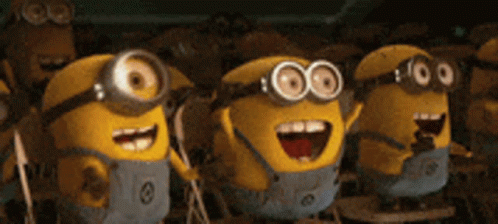
That's how you add sign in and sign out modern UI using Firebase in Python in the simplest way!
Languages and Tools
Contact
🌵 Stay peace and keep coding!
Screenshots of the UI
Project details
Release history Release notifications | RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distribution
Hashes for firebase_auth_ui-0.0.3-py3-none-any.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | 2ad7e06d0ce78af94e9628af4dff1ac4cfe6c4f30ebf73c19e9bdc7717bbc30e |
|
MD5 | da3269729a6f9126753612e594b09e7d |
|
BLAKE2b-256 | 6b60238302c181811db5e9b5e4f6d37307fd07baedcb369111fc080a02198f1d |