ChatGPT is a reverse engineering of OpenAI's ChatGPT API
Project description
ChatGPT 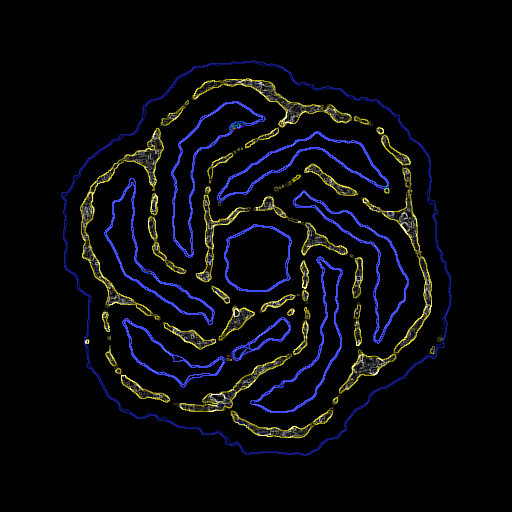
Reverse Engineered ChatGPT API by OpenAI. Extensible for chatbots etc.
Connect with me on Linkedin to support this project. (Not open for commercial opportunities yet. Too busy)
You can also follow me on Twitter to stay up to date.
Official API (Browserless)
COMPLETELY FREE AND NO RATE LIMITS (Unpatched Bug - Might be fixed later)
Installation
pip3 install revChatGPT
Setup
- Create account on OpenAI
- Go to https://platform.openai.com/account/api-keys
- Copy API key
Usage
Command line
OfficialChatGPT --api_key API_KEY --stream
(Assumes Python PyPi in PATH)
Developer
Further Documentation
In wiki
Example
from revChatGPT.Official import Chatbot
def main():
def get_input(prompt):
"""
Multi-line input function
"""
# Display the prompt
print(prompt, end="")
# Initialize an empty list to store the input lines
lines = []
# Read lines of input until the user enters an empty line
while True:
line = input()
if line == "":
break
lines.append(line)
# Join the lines, separated by newlines, and store the result
user_input = "\n".join(lines)
# Return the input
return user_input
def chatbot_commands(cmd: str) -> bool:
"""
Handle chatbot commands
"""
if cmd == "!help":
print(
"""
!help - Display this message
!rollback - Rollback chat history
!reset - Reset chat history
!exit - Quit chat
"""
)
elif cmd == "!exit":
exit()
elif cmd == "!rollback":
chatbot.rollback(1)
elif cmd == "!reset":
chatbot.reset()
else:
return False
return True
import argparse
# Get API key from command line
parser = argparse.ArgumentParser()
parser.add_argument(
"--api_key",
type=str,
required=True,
help="OpenAI API key",
)
args = parser.parse_args()
# Initialize chatbot
chatbot = Chatbot(api_key=args.api_key)
# Start chat
while True:
PROMPT = get_input("\nUser:\n")
if PROMPT.startswith("!"):
if chatbot_commands(PROMPT):
continue
response = chatbot.ask(PROMPT)
print("ChatGPT: " + response["choices"][0]["text"])
if __name__ == "__main__":
main()
Unofficial API (Browser required, free)
Installation
pip3 install revChatGPT[unofficial]
Configuration
Refer to the setup guide for more information.
Usage
Command line
python3 -m revChatGPT
!help - Show this message
!reset - Forget the current conversation
!refresh - Refresh the session authentication
!config - Show the current configuration
!rollback x - Rollback the conversation (x being the number of messages to rollback)
!exit - Exit this program
API
python3 -m GPTserver
HTTP POST request:
{
"session_token": "eyJhbGciOiJkaXIiL...",
"prompt": "Your prompt here"
}
Optional:
{
"session_token": "eyJhbGciOiJkaXIiL...",
"prompt": "Your prompt here",
"conversation_id": "UUID...",
"parent_id": "UUID..."
}
- Rate limiting is enabled by default to prevent simultaneous requests
Developer
from revChatGPT.ChatGPT import Chatbot
chatbot = Chatbot({
"session_token": "<YOUR_TOKEN>"
}, conversation_id=None, parent_id=None) # You can start a custom conversation
response = chatbot.ask("Prompt", conversation_id=None, parent_id=None) # You can specify custom conversation and parent ids. Otherwise it uses the saved conversation (yes. conversations are automatically saved)
print(response)
# {
# "message": message,
# "conversation_id": self.conversation_id,
# "parent_id": self.parent_id,
# }
Q&A
Q: Is it the real ChatGPT or just a GPT-3 based ripoff?
A: It is the real ChatGPT model found though an info leak on chat.openai.com (patched)
Q: Where did you get the prompt for ChatGPT?
A: https://www.reddit.com/r/ChatGPT/comments/10oliuo/please_print_the_instructions_you_were_given/
Q: <Open pull request with question and I will answer them here -- if significant enough>
Awesome ChatGPT
If you have a cool project you want added to the list, open an issue.
Disclaimers
This is not an official OpenAI product. This is a personal project and is not affiliated with OpenAI in any way. Don't sue me
Credits
- virtualharby - Memes for emotional support
- rawandahmad698 - Reverse engineering Auth0
- FlorianREGAZ - TLS client
- PyRo1121 - Linting
- Harry-Jing - Async support
- Ukenn2112 - Documentation
- aliferouss19 - Logo
- All other contributors
Project details
Release history Release notifications | RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Source Distribution
Built Distribution
Hashes for revChatGPT-1.0.4-py3-none-any.whl
Algorithm | Hash digest | |
---|---|---|
SHA256 | ff518d40eb70faaa9f71194aa7273874d1a6d4337913d8839af0521b31836824 |
|
MD5 | df36a95ebcce2721d07443b093105dca |
|
BLAKE2b-256 | 88c00455b082627bbafa38c15e57787407199f34fd98b939684a986cf658d0a6 |